How to Convert Online Forms to Fillable PDF Documents
Follow this tutorial to learn how to create a sample job application HTML form using the SurveyJS Form Library. Export the HTML form as a fillable PDF, ready to be saved directly on your device.
Why Build Fillable PDF Forms
Fillable PDF forms allow companies to accommodate diverse user preferences and cater for a broader audience, including users without internet access or those who like working offline. Since PDF forms can be completed and submitted offline, they are ideal for situations when internet connectivity is limited or unreliable, such as during field work or in remote locations. Form builders that support export of web forms to fillable, read-only, or completed PDF forms offer companies enhanced flexibility and accessibility and streamline data collection and processing.
Additionally, PDF forms preserve the intended layout, ensuring that printed or fillable forms accurately represent their online counterparts.
Live Demo
Create a Job Application Form
A job application form is commonly used by businesses and organizations to gather information from jobseekers. It typically includes fields for personal information, educational background, work experience, and additional questions specific to the job role or company.
In our sample form, we will include the following sections:
- Personal Information:
- Full Name
- Date of Birth
- Address
- Contact Information
- Phone Number
- Email Address
- Job-specific questions
- Position Applied
- Expected Salary
- Relevant skills for a selected job position
- A Signature field to confirm data validity
With our PDF Form Builder, you can build an HTML form and then convert it into a fillable PDF file.
View Free Demo
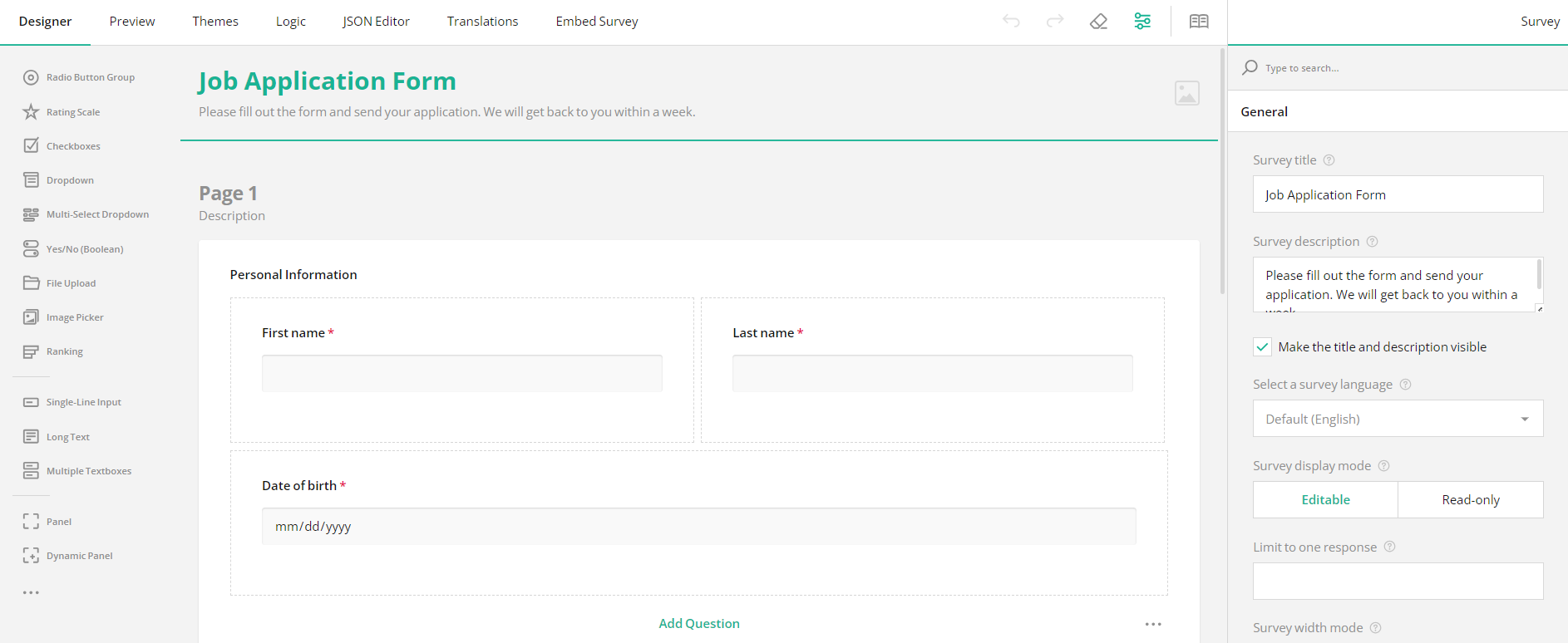
To starting building a job application form, you need to add SurveyJS Form Library to your application. Form Library supports React, Angular, jQuery, Knockout, and Vue.js. In this blog post, we'll integrate SurveyJS Form Library into a React application. To find tutorials for other frameworks, refer to the Get Started help topics.
Install the survey-react-ui
npm package to add SurveyJS Form Library to your React application:
npm install survey-react-ui --save
In SurveyJS, a form's content is represented by a form JSON schema. The following code shows a JSON schema for a job application form:
View Form JSON Schema
// surveyJSON.js
export const surveyJson = {
title: 'Job Application Form',
description:
'Please fill out the form and send your application. We will get back to you within a week.',
pages: [
{
name: 'personal-info-page',
elements: [
{
type: 'panel',
name: 'personal-info-panel',
elements: [
{
type: 'text',
name: 'first-name',
title: 'First name',
isRequired: true,
},
{
type: 'text',
name: 'last-name',
startWithNewLine: false,
title: 'Last name',
isRequired: true,
},
{
type: 'text',
name: 'birthdate',
title: 'Date of birth',
isRequired: true,
inputType: 'date',
},
],
title: 'Personal Information',
},
{
type: 'panel',
name: 'location-panel',
elements: [
{
type: 'dropdown',
name: 'country',
title: 'Country',
choicesByUrl: {
url: 'https://surveyjs.io/api/CountriesExample',
},
},
{
type: 'text',
name: 'city',
title: 'City/Town',
},
{
type: 'text',
name: 'zip',
startWithNewLine: false,
title: 'Zip Code',
validators: [
{
type: 'numeric',
},
],
maskType: 'pattern',
maskSettings: {
pattern: '9999',
},
},
{
type: 'text',
name: 'address',
title: 'Street Address',
},
],
title: 'Your Location',
},
{
type: 'panel',
name: 'panel2',
elements: [
{
type: 'text',
name: 'email',
title: 'Email',
inputType: 'email',
placeholder: 'mail@example.com',
},
{
type: 'text',
name: 'phone',
startWithNewLine: false,
title: 'Phone',
maskType: 'pattern',
maskSettings: {
saveMaskedValue: true,
pattern: '+999 99 999999',
},
placeholder: '(000) 000-0000',
},
],
title: 'Contact Information',
},
{
type: 'panel',
name: 'panel3',
elements: [
{
type: 'dropdown',
name: 'position',
title: 'What position are you applying for?',
choices: [
{
value: 'frontend',
text: 'Frontend Developer',
},
{
value: 'backend',
text: 'Backend Developer',
},
{
value: 'fullstack',
text: 'Full-Stack Developer',
},
{
value: 'intern',
text: 'Intern',
},
],
},
{
type: 'text',
name: 'salary',
startWithNewLine: false,
title: 'Expected salary (in US dollars)',
validators: [
{
type: 'numeric',
},
],
inputType: 'number',
},
{
type: 'comment',
name: 'experience',
title: 'What relevant skills or experience do you have?',
},
{
type: 'signaturepad',
name: 'signature',
title: 'Signature',
isRequired: true,
placeholder: 'Please sign here. ',
},
],
},
],
},
],
showQuestionNumbers: 'off',
questionErrorLocation: 'bottom',
completeText: 'Send',
widthMode: 'responsive',
}
Create a React component that would render this form JSON schema:
// SurveyComponent.jsx
import React, { useCallback } from 'react';
import 'survey-core/defaultV2.min.css';
import { Model } from 'survey-core';
import { Survey } from 'survey-react-ui';
import { surveyJson } from './surveyJSON';
function SurveyComponent() {
const survey = new Model(surveyJson);
const printResults = useCallback((sender) => {
const results = JSON.stringify(sender.data);
console.log(results);
}, []);
survey.onComplete.add(printResults);
return <Survey model={survey} />;
}
export default SurveyComponent;
A job application form is now rendered on screen. Respondents can fill in form fields and submit the form.
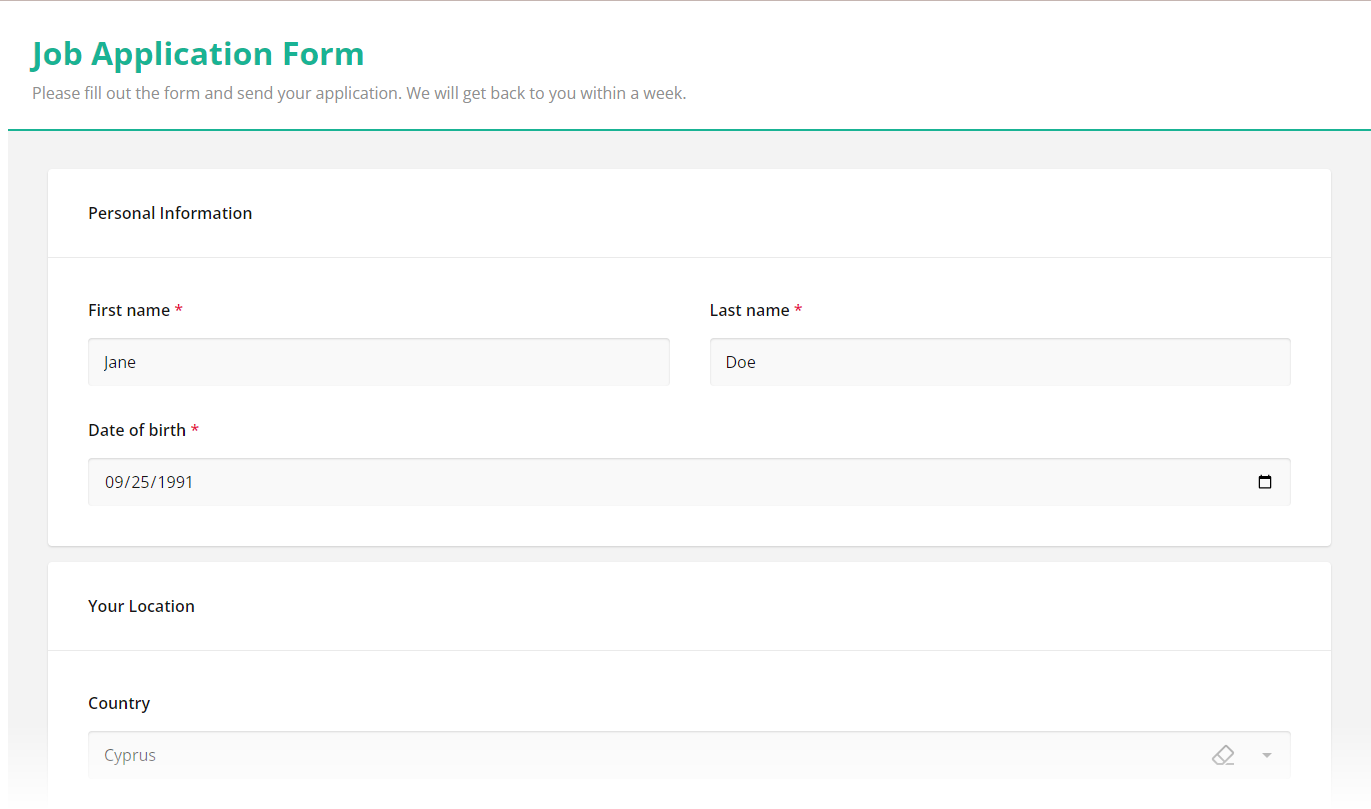
Build a PDF Form
To create a PDF form based on the supplied form JSON schema, use SurveyJS PDF Generator. To add this library to a React application, install the survey-pdf
npm package. If you want to learn how to integrate SurveyJS PDF Generator into an Angular, Knockout, jQuery, or Vue.js application, visit the corresponding Get Started documentation section.
npm install survey-pdf --save
Implement a function that creates a SurveyPDF
object instance. The SurveyPDF
object constructor accepts a survey JSON schema and an object with PDF export options. These options include page orientation, paper size and format, margin, and other settings. For more information on available PDF export options, visit the PDF Form Settings help topic.
To populate a PDF document with user responses, assign a response object to the data
property of a SurveyPDF
object instance. To download a PDF document on a client, call SurveyPDF
's save()
method and pass the target file name.
function saveSurveyToPdf(filename, formJson, userResponse) {
const options = {
fontSize: 14,
margins: {
left: 10,
right: 10,
top: 10,
bot: 10,
},
format: [210, 297],
};
const surveyPDF = new SurveyPDF(formJson, options);
surveyPDF.data = userResponse;
surveyPDF.save(filename);
}
Now that you have a function that saves a PDF document, you need a UI element that would allow respondents to easily produce a fillable PDF form. For example, you can add a custom "Save as PDF" button to the navigation bar below the form.
// ...
import { Model } from "survey-core";
function saveSurveyToPdf(filename, formJson, userResponse) {
// ...
}
function SurveyComponent() {
const survey = new Model(surveyJson);
// ...
survey.addNavigationItem({
id: "survey_save_as_file",
title: "Save as PDF",
action: () => {
saveSurveyToPdf("jobApplication.pdf", surveyJson, survey.data);
}
});
return <Survey model={survey} />;
}
export default SurveyComponent;
The resulting editable PDF form appears as follows. A PDF document uses acro-forms to represent editable form fields.
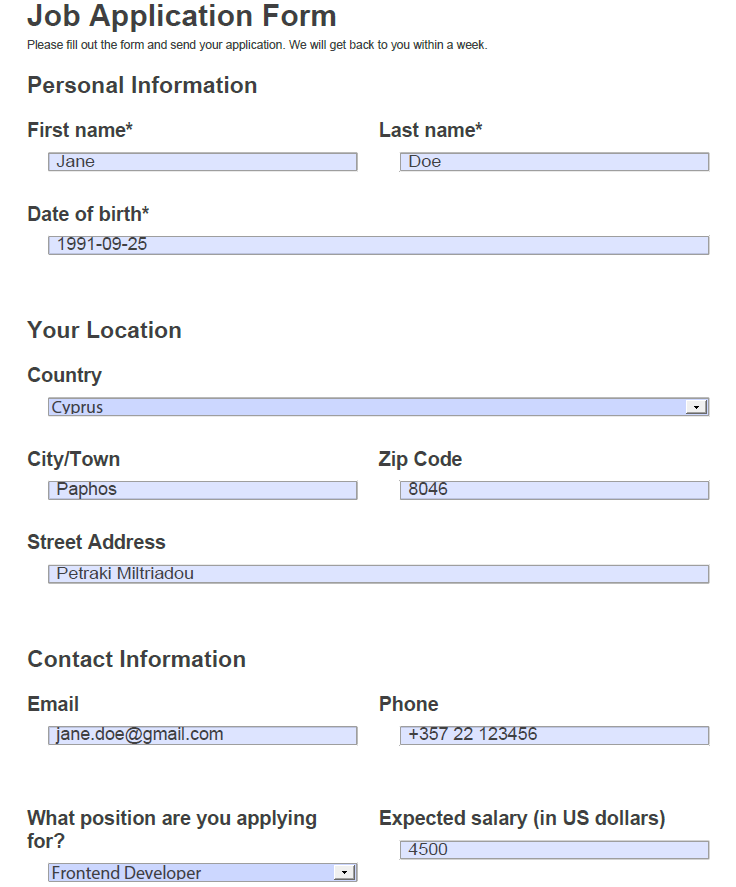
View Full Survey Component Code
import React, { useCallback } from 'react';
import 'survey-core/defaultV2.min.css';
import { Model } from 'survey-core';
import { Survey } from 'survey-react-ui';
import { surveyJson } from './surveyJSON';
import { userResponse } from './response';
import { SurveyPDF } from 'survey-pdf';
function saveSurveyToPdf(filename, formJson, userResponse) {
let options = {
fontSize: 14,
margins: {
left: 10,
right: 10,
top: 10,
bot: 10,
},
format: [210, 297],
};
const surveyPDF = new SurveyPDF(formJson, options);
surveyPDF.data = userResponse;
surveyPDF.save(filename);
}
function SurveyComponent() {
const survey = new Model(surveyJson);
survey.data = userResponse;
survey.addNavigationItem({
id: 'survey_save_as_file',
title: 'Save as PDF',
action: () => {
saveSurveyToPdf('jobApplication.pdf', surveyJson, survey.data);
},
});
const printResults = useCallback((sender) => {
const results = JSON.stringify(sender.data);
console.log(results);
}, []);
survey.onComplete.add(printResults);
return <Survey model={survey} />;
}
export default SurveyComponent;
Save PDF Documents as Blob, Base64 URL, or Raw PDF
Depending on your use case and requirements, you may consider storing PDF files as Blob, Base64 URL, or Raw PDF.
Blob
Efficient for direct database storage without encoding, simplifying data management and retrieval.Base64 URL
Useful for web applications to embed PDF content in HTML or other formats, ensuring safe transmission over text-based protocols like HTTP.Raw PDF
Maintains original file integrity, allowing direct access without encoding or decoding overhead.
For more information on how to generate PDF documents in different formats, view the following demo: