Web Form Navigation System: The Ultimate Guide (Part 1)
SurveyJS ships with a variety of built-in navigation features that you can implement in your forms to simplify the filling-out process. In this article, we will talk about the types of navigation features that are available out of the box and how to configure them. In the second part, we will show how to create custom navigation components if something more sophisticated is required.
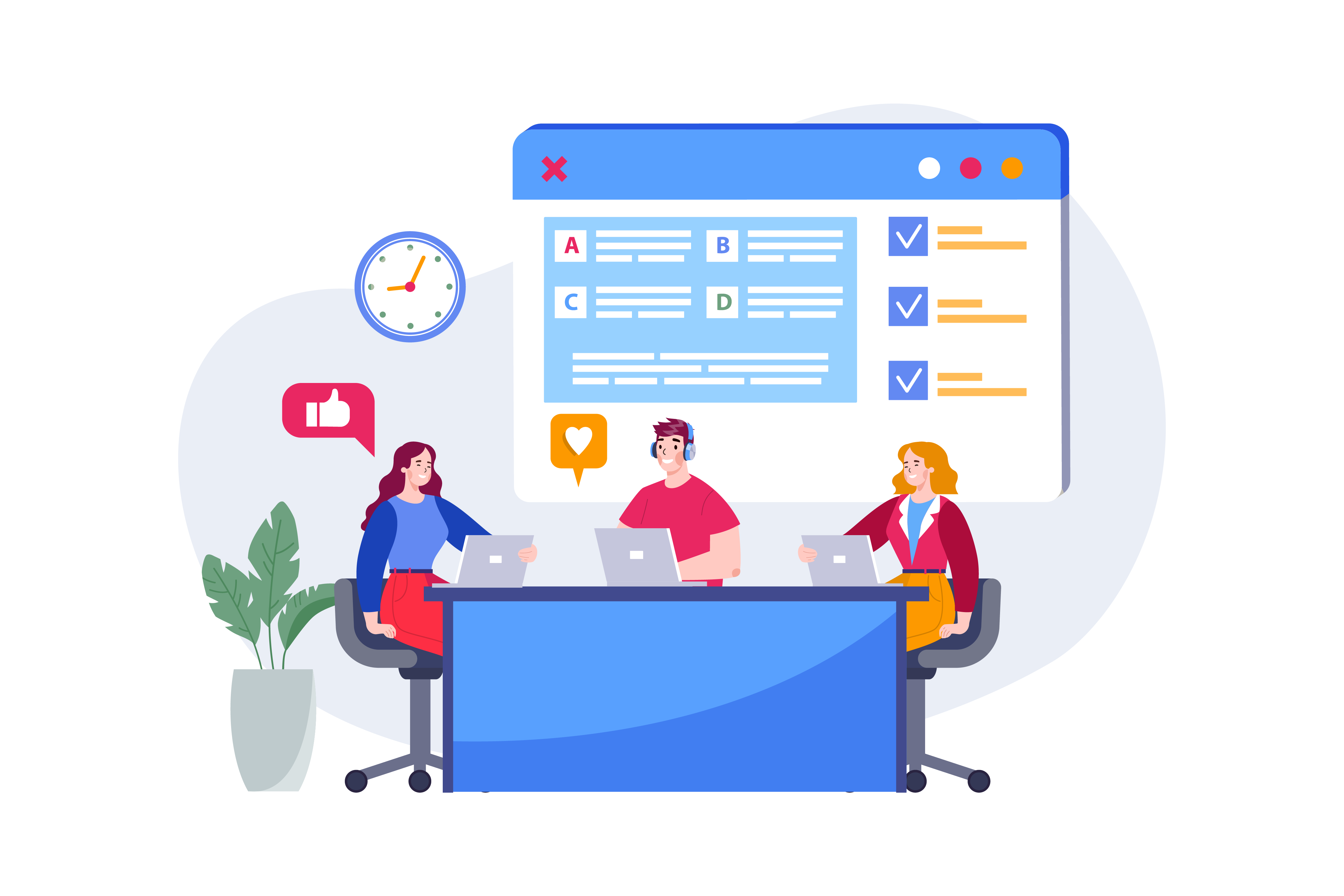
Why Implement a Navigation System in a Web Form
Having navigation elements in a web form or survey is incredibly convenient for both a respondent and a creator of a form. For the respondent, having a clear and easy-to-use navigation system in place makes filling out the form or survey much simpler and less time-consuming. Rather than having to scroll through a long, complex form in one sitting, users can navigate to specific sections or pages that they need to fill out, making the process feel less overwhelming. Additionally, if the user needs to go back to change a certain answer or review their responses, a navigation system makes it easy to do so, thus greatly enhancing the user experience.
For the creator of the form or survey, navigation can help ensure that users complete all required sections or questions. By breaking up the form into manageable sections, creators can also help users stay engaged and more likely to complete the form, which improves the overall quality of the data collected.
Form Navigation Types
With SurveyJS Form Library, you can implement all popular types of form navigation:
Progress indicators
Progress indicators show users how far along they are in the form or survey completion process. They can be in the form of a progress bar, percentage, or number of pages completed out of the total.Tabs
Tabs break up a form or survey into multiple sections, usually dedicated to a particular topic ("Contact information", "Symptoms", etc.). Each section appears in its own tab. Users can click on a tab to navigate to the section they want to complete.Previous/Next buttons
Previous/Next buttons allow users to move forward or backward through a form or survey, usually between pages or questions (if only one question is shown at once). These buttons can be used in combination with other navigation types.Jump links
Jump links allow users to skip to a specific question, section, or page of a form or survey. They are useful when users want to navigate to a certain question to change an answer or simply to review questions of the entire section before answering them.Side navigation (TOC)
Side navigation appears on the side of a form or survey and shows a list of all form questions, sections, or pages. It can be used in combination with other navigation types to provide users with multiple ways to navigate through the form or survey.
Now let's take a closer look at how to configure each type using the built-in navigation functionality of SurveyJS Form Library.
JSON as a Common Ground
SurveyJS uses the industry-standard JSON format to define a form as a data model that describes its layout and contents. In order to configure the navigation system in a form, we will mostly be dealing with following two classes:
SurveyModel
- Contains properties and methods that allow you to control the survey and access its elements.PageModel
- Describes a survey page and contains properties and methods that allow you to control the page and access its elements (panels and questions).
Table of Contents
Let's start with a Table of Contents (TOC). As an example, we will add a TOC to a complex multipage Patient Assessment Form, which has seven different sections, each dedicated to a particular topic: "Patient Information", "Health History", "Recent Hospitalizations", etc. To manage TOC visibility, we need to enable or disable the showTOC
property by assigning the true
or false
Boolean values to it. Since this is a property of a SurveyModel
object, we'll specify it at the bottom or the top of the JSON, not within the configuration of a page or its elements (panels or questions):
export const json = {
"title": "Patient Assessment Form",
// ...
"showTOC": true,
"pages": [
{
"name": "Patient information",
"title": "Patient information",
"elements": [
{
"type": "panel",
"name": "patient-information",
"title": "All fields with an asterisk (*) are required fields and must be filled out in order to process the information in strict confidentiality.",
"elements": [
{
"type": "text",
"name": "first-name",
"title": "First name",
"isRequired": true
},
// ...
You can also decide on the location of a TOC by assigning the "left"
or "right"
string values to the tocLocation
property. When you generate the form JSON definition using a no-code UI of your in-house Survey Creator (please refer to its free full-scale demo to learn more) and enable a TOC in its Property Grid, the TOC is positioned on the left by default.
export const json = {
"title": "Patient Assessment Form",
// ...
"showTOC": true,
"tocLocation": "right",
"pages": [
{
"name": "Patient information",
"title": "Patient information",
// ...
A TOC displays page titles or names if the titles are undefined. If both a page title and name are specified, the title
property overrides the name
property. In order to modify the titles of the TOC, you can define them for each page using the navigationTitle
property. navigationTitle
overrides the title
and name
properties if either or both are also specified.
export const json = {
"title": "Patient Assessment Form",
// ...
"showTOC": true,
"tocLocation": "right",
"pages": [
{
"name": "Patient information",
"navigationTitle": "Nice TOC title",
"title": "Patient information",
// ...
Here is how a TOC appears after the configuration described above have been applied.
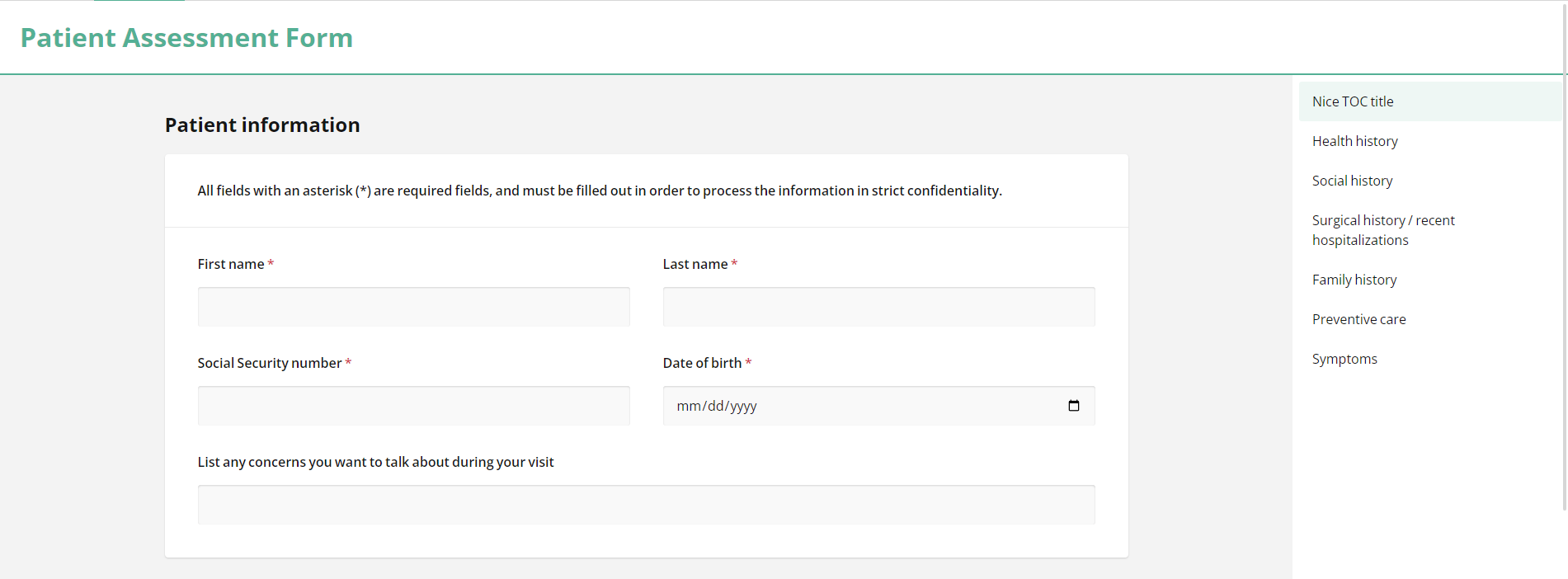
Progress Bar
The easiest way to show users how far along they are in the form or survey completion process is to display a basic progress bar. Enable the showProgressBar
property and assign "aboveHeader"
, "belowHeader"
"bottom"
, or "topBottom"
string values to the progressBarLocation
property to align the progress bar within your form or survey. By default, the progress bar displays how many pages are completed out of the total number of pages.
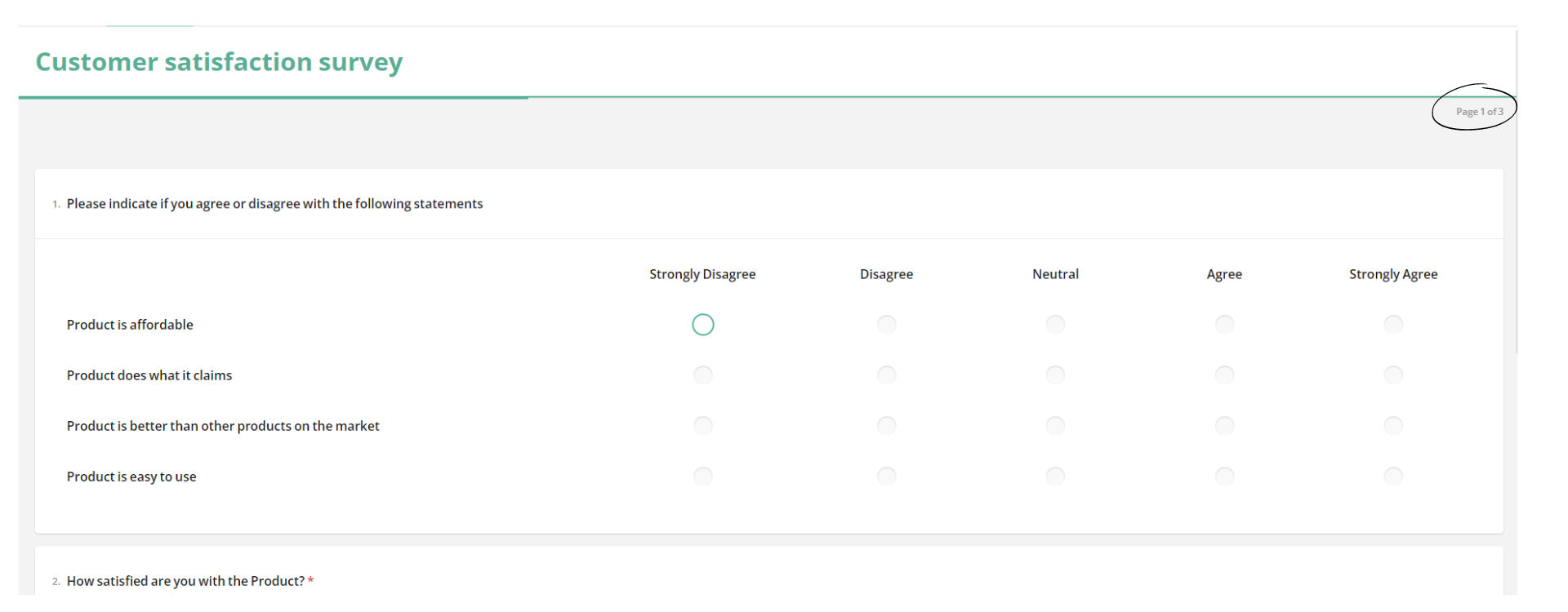
To specify the information the progress bar should display, set the progressBarType
property to one of the following string values:
"pages"
"questions"
"requiredQuestions"
"correctQuestions"
"buttons"
The first two values are quite self-explanatory. The "requiredQuestions"
value displays the number of completed questions that require an answer out of the total number of such mandatory questions. The "correctQuestions"
value displays the number of correct answers out of the total number of all questions in a form. To use this progress bar type, you need to make sure that the correctAnswer
property is configured for each question.
If you want to learn more about SurveyJS features for quizzes and tests, refer to the dedicated guide: How to Create an Online Quiz or Assessment test using SurveyJS.
If your survey or form has several pages, you can set the progressBarType
property to "buttons"
to turn the progress bar into a navigation control. Add a navigationTitle
property with a desired string value to each page. Users can click navigation titles to switch between pages. This is how the progress bar with navigation appears to a user:
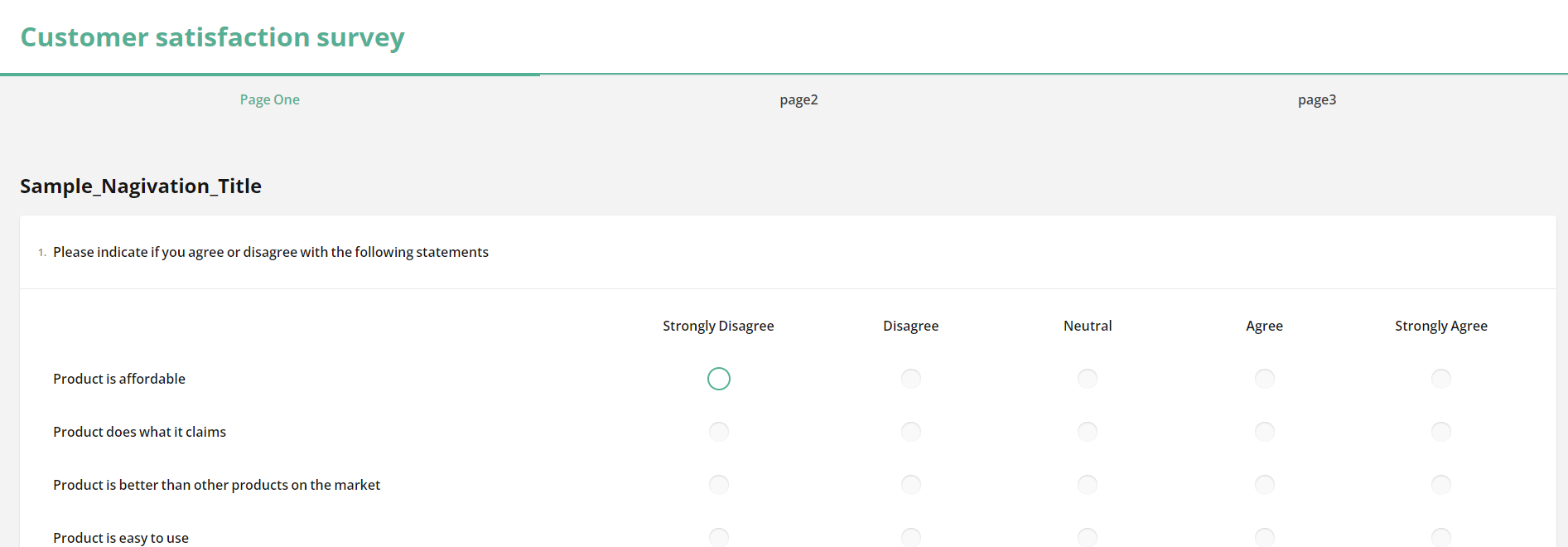
Here is the navigation bar configuration in JSON:
export const json = {
"title": "Customer satisfaction survey",
"showProgressBar": true,
"progressBarType": "buttons",
"pages": [
{
"navigationTitle": "Page One",
"elements": [
{
"type": "matrix",
"name": "Quality",
"title": "Please indicate if you agree or disagree with the following statements",
// ...
View Demo: Buttons Progress Bar and Navigation
Previous/Next Buttons
Respondents can use Next, Previous, and Complete buttons to complete a form or survey and move forward or backward through it. These buttons are displayed at the bottom of your survey. If you want to change their location, set the navigationButtonsLocation
property to "top"
or "topBottom"
.
Disable the showNavigationButtons
property to hide the buttons. In this case, enable the autoAdvanceEnabled
property so that respondents can automatically move to the next question or page once they provided answers on the current one. Please note that if you enable this property, the survey is also completed automatically. Set the autoAdvanceAllowComplete
property to false
if you want to disable this behavior.
export const json = {
// ...
"showNavigationButtons": false,
"autoAdvanceEnabled": true,
"autoAdvanceAllowComplete": false,
"pages": [
// ...
If you want to show the buttons only on some pages, enable the showNavigationButtons
property for those pages.
To prevent users from going backward to the previous answer, disable the showPrevButton
property. This setting hides the Previous button.
SurveyJS allows you to use the following SurveyModel
properties to modify the default captions of navigation buttons:
Form Preview
To allow respondents to preview answers before they are submitted, enable the showPreviewBeforeComplete
property. By default, the preview page displays all visible questions. If it should display only answered questions, change the previewMode
property value to "answeredQuestions"
.
View Demo: Show Preview Before Complete
If you'd like to implement custom navigation functionality in your forms (for example, add a button that enables users to clear their answers or add a custom progress bar component), stay tuned for the second part of the guide, which will be devoted to the configuration of custom navigation elements.