SurveyCreatorModel
A class with properties, methods, and events that allow you to configure Survey Creator and manage its elements.
Inherited from the following class(es):
Properties
Methods
Events
Gets or sets the currently displayed tab.
Possible values:
- Type:
- string writable
- Implemented in:
- SurveyCreatorModel
- See also:
- makeNewViewActive
Adds new items to the pages
, triggers
, calculatedValues
, and completedHtmlOnCondition
arrays in the existing survey JSON schema.
Use this method to merge the collection properties of two survey JSON schemas:
import { SurveyCreatorModel } from "survey-creator-core";
const creatorOptions = { ... };
const creator = new SurveyCreatorModel(creatorOptions);
const surveyJson1 = { ... };
const surveyJson2 = { ... };
creator.JSON = surveyJson1;
creator.addCollectionItemsJson(surveyJson2);
// `creator.JSON` contains the merged survey JSON schema
- Type:
- (json: any, insertPageIndex?: number) => void
- Parameters:
-
json, type: any ,
A JSON object that contains the
pages
,triggers
,calculatedValues
, and/orcompletedHtmlOnCondition
array(s).insertPageIndex, type: number ,A zero-based index at which to insert new pages.
- Implemented in:
- SurveyCreatorModel
Specifies where to add new questions when users click the "Add Question" button.
Accepted values:
true
(default)
New questions are added to the end of a survey page.false
New questions are added after the currently selected question on the design surface.
- Type:
- boolean readonly
- Implemented in:
- SurveyCreatorModel
Adds a custom tab to Survey Creator.
- Type:
- (name: string, plugin: ICreatorPlugin, title?: string, componentName?: string, index?: number) => void
- Parameters:
-
name, type: string ,
A unique tab ID.
plugin, type: ICreatorPlugin ,An object that allows you to handle user interactions with the tab.
title, type: string ,A tab caption. If
title
is undefined, thename
argument value is displayed instead. To localize the caption, add its translations to theed
object within localization dictionaries and passed.propertyName
as thetitle
argument.componentName, type: string ,The name of the component that renders tab markup. Default value:
"svc-tab-" + name
.index, type: number ,A zero-based index that specifies the tab's position relative to other tabs.
- Implemented in:
- SurveyCreatorModel
Specifies whether users can switch between UI themes in the Preview tab.
Default value: true
- Type:
- boolean readonly
- Implemented in:
- SurveyCreatorModel
- See also:
- themeForPreview
Specifies whether users can edit expressions in the Logic tab as plain text.
If you set this property to false
, users can only use UI elements to edit logical expressions.
Default value: true
- Type:
- boolean readonly
- Implemented in:
- SurveyCreatorModel
- See also:
- showLogicTab
Specifies whether users can add, edit, and delete survey pages.
Default value: true
- Type:
- boolean writable
- Implemented in:
- SurveyCreatorModel
- See also:
- pageEditMode
A delay between changing survey settings and saving the survey JSON schema, measured in milliseconds. Applies only when the isAutoSave
property is true
.
Default value: 500 (inherited from settings.autoSave.delay
)
If a user changes the settings once again during the delay, only the latest version will be saved.
- Type:
- number readonly
- Implemented in:
- SurveyCreatorModel
Opens a dialog window for users to select files.
- Type:
- (input: HTMLInputElement, callback: (files: {}) => void, context?: { element: Base; item?: any; elementType?: string; propertyName?: string; }) => void
- Parameters:
-
callback, type: (files: {}) => void ,
A callback function that you can use to process selected files. Accepts an array of JavaScript File objects.
context, type: { element: Base; item?: any; elementType?: string; propertyName?: string; }
- Implemented in:
- SurveyCreatorModel
- See also:
- onOpenFileChooser * , onUploadFile
Creates a new object that has the same type and properties as the current SurveyJS object.
- Type:
- () => Base
- Implemented in:
- Base
Collapses all categories in Property Grid.
- Type:
- () => void
- Implemented in:
- SurveyCreatorModel
- See also:
- expandAllPropertyGridCategories
Collapses a specified category in Property Grid.
- Type:
- (name: string) => void
- Parameters:
- Implemented in:
- SurveyCreatorModel
- See also:
- expandPropertyGridCategory
Creates a copy of a specified page and inserts the copy next to this page.
- Type:
- (page: PageModel) => PageModel
- Parameters:
- Return Value:
- Implemented in:
- SurveyCreatorModel
- See also:
- onPageAdding * , onPageAdded
Deletes a survey element: a question, panel, or page.
If you want to delete the focused element, pass the selectedElement
property value to this method.
- Type:
- (element: Base) => void
- Parameters:
-
element, type: Base ,
A survey element to delete.
- Implemented in:
- SurveyCreatorModel
- See also:
- onElementDeleting
Deletes all custom translation strings for a specified locale from Survey Creator and from the generated survey JSON schema.
- Type:
- (locale: string) => void
- Parameters:
-
locale, type: string ,
A locale code (for example, "en").
- Implemented in:
- SurveyCreatorModel
- See also:
- locale
Expands all categories in Property Grid.
- Type:
- () => void
- Implemented in:
- SurveyCreatorModel
- See also:
- collapseAllPropertyGridCategories
Expands a specified category in Property Grid.
- Type:
- (name: string) => void
- Parameters:
- Implemented in:
- SurveyCreatorModel
- See also:
- collapsePropertyGridCategory
Creates a copy of a specified question, inserts the copy next to this question, and (optionally) selects it on the design surface.
- Type:
- (question: Base, selectCopy?: boolean) => IElement
- Parameters:
-
question, type: Base ,
A question to copy.
selectCopy, type: boolean ,(Optional) Pass
true
if you want to select the copy on the design surface. Default value:false
.
- Return Value:
-
The instance of a new question.
- Implemented in:
- SurveyCreatorModel
Assigns a new configuration to the current SurveyJS object. This configuration is taken from a passed JSON object.
The JSON object should contain only serializable properties of this SurveyJS object. Event handlers and properties that do not belong to the SurveyJS object are ignored.
- Type:
- (json: any, options?: ILoadFromJSONOptions) => void
- Parameters:
-
json, type: any ,
A JSON object with properties that you want to apply to the current SurveyJS object.
options, type: ILoadFromJSONOptions ,An object with configuration options.
- Implemented in:
- Base
- See also:
- toJSON
Returns a JsonObjectProperty
object with metadata about a serializable property that belongs to the current SurveyJS object.
If the property is not found, this method returns null
.
- Type:
- (propName: string) => JsonObjectProperty
- Parameters:
-
propName, type: string ,
A property name.
- Implemented in:
- Base
Returns the value of a property with a specified name.
If the property is not found or does not have a value, this method returns either undefined
, defaultValue
specified in the property configuration, or a value passed as the defaultValue
parameter.
- Type:
- (name: string, defaultValue?: any) => any
- Parameters:
-
name, type: string ,
A property name.
defaultValue, type: any ,(Optional) A value to return if the property is not found or does not have a value.
- Implemented in:
- Base
Returns true if initial survey was empty. It was not set via JSON property and default new survey is empty as well.
- Type:
- (json: any, clearState: boolean) => void
- Parameters:
-
json, type: anyclearState, type: boolean
- Return Value:
-
true if initial survey doesn't have any elements or properties
- Implemented in:
- SurveyCreatorModel
Allows users to edit choice values instead of choice texts on the design surface.
Default value: false
(users edit choice texts)
If you enable this property, users cannot edit choice texts because the Property Grid hides the Text column for choices, rate values, columns and rows in Single-Select Matrix, and rows in Multi-Select Matrix questions.
- Type:
- boolean readonly
- Implemented in:
- SurveyCreatorModel
- See also:
- showObjectTitles
Returns true
if the object is included in a survey.
This property may return false
, for example, when you create a survey model dynamically.
- Type:
- boolean readonly
- Implemented in:
- Base
Specifies whether to automatically save a survey or theme JSON schema each time survey or theme settings are changed.
Default value: false
If you enable this property, Survey Creator calls the saveSurveyFunc
or saveThemeFunc
function to save the survey or theme JSON schema. The schemas are saved with a 500-millisecond delay after users change settings. You can specify the autoSaveDelay
property to increase or decrease the delay.
- Type:
- boolean readonly
- Implemented in:
- SurveyCreatorModel
Returns true
if the survey is being designed in Survey Creator.
- Type:
- boolean readonly
- Implemented in:
- Base
Returns true
if the object configuration is being loaded from JSON.
- Type:
- boolean readonly
- Implemented in:
- Base
Returns true
if an undo or redo operation is in progress.
- Type:
- boolean readonly
- Implemented in:
- SurveyCreatorModel
Specifies whether to enable support for right-to-left languages.
Default value: false
- Type:
- boolean writable
- Implemented in:
- SurveyCreatorModel
Returns true
if a passed value
is an empty string, array, or object or if it equals to undefined
or null
.
- Type:
- (value: any, trimString?: boolean) => boolean
- Parameters:
-
value, type: any ,
A value to be checked.
trimString, type: boolean ,(Optional) When this parameter is
true
, the method ignores whitespace characters at the beginning and end of a string value. Passfalse
to disable this functionality.
- Implemented in:
- Base
A survey JSON schema.
This property allows you to get or set the JSON schema of a survey being configured. Alternatively, you can use the text
property.
- Type:
- any writable
- Implemented in:
- SurveyCreatorModel
Specifies the locale of the Survey Creator UI.
Default value: ""
(inherited from editorLocalization.currentLocale
)
- Type:
- string writable
- Implemented in:
- SurveyCreatorModel
Switches the active tab. Returns false
if the tab cannot be switched.
- Type:
- (tabName: string) => boolean
- Parameters:
-
tabName, type: string ,
A tab that you want to make active:
"designer"
,"test"
,"theme"
,"editor"
,"logic"
, or"translation"
.
- Return Value:
-
false
if the active tab cannot be switched,true
otherwise.
- Implemented in:
- SurveyCreatorModel
Limits the number of choices that users can add to Checkbox, Dropdown, and Radiogroup questions.
Default value: 0 (unlimited, taken from settings.propertyGrid.maximumChoicesCount
)
- Type:
- number readonly
- Implemented in:
- SurveyCreatorModel
Limits the number of columns that users can add to Matrix, Matrix Dynamic, and Matrix Dropdown questions.
Default value: 0 (unlimited, taken from settings.propertyGrid.maximumColumnsCount
)
- Type:
- number readonly
- Implemented in:
- SurveyCreatorModel
Limits the number of rate values that users can add to Rating questions.
Default value: 0 (unlimited, taken from settings.propertyGrid.maximumRateValues
)
- Type:
- number readonly
- Implemented in:
- SurveyCreatorModel
Limits the number of rows that users can add to Matrix and Matrix Dropdown questions.
Default value: 0 (unlimited, taken from settings.propertyGrid.maximumRowsCount
)
- Type:
- number readonly
- Implemented in:
- SurveyCreatorModel
Limits the number of items in a logical condition.
Default value: -1 (unlimited)
- Type:
- number readonly
- Implemented in:
- SurveyCreatorModel
Limits the number of nested panels within a Panel element.
Default value: -1 (unlimited)
- Type:
- number readonly
- Implemented in:
- SurveyCreatorModel
Limits the number of visible choices. Users can click "Show more" to view hidden choices.
Set this property to -1 if you do not want to hide any choices.
Default value: 10
- Type:
- number readonly
- Implemented in:
- SurveyCreatorModel
Limits the minimum number of choices in Checkbox, Dropdown, and Radiogroup questions. Set this property if users should not delete choices below the specified limit.
Default value: 0 (unlimited, taken from settings.propertyGrid.minimumChoicesCount
)
- Type:
- number readonly
- Implemented in:
- SurveyCreatorModel
Displays a toast notification with a specified message.
If you want to implement custom toast notification from scratch, handle the onNotify
event.
- Type:
- (message: string, type?: "info" | "error") => void
- Parameters:
-
message, type: string ,
A message to display.
type, type: "info" | "error" ,A notification type:
"info"
(default) or"error"
.
- Implemented in:
- SurveyCreatorModel
An event that is raised after the active tab is switched.
Parameters:
sender
:SurveyCreatorModel
A Survey Creator instance that raised the event.options.tabName
:string
A tab that has become active:"designer"
,"test"
,"theme"
,"editor"
,"logic"
, or"translation"
.
- Type:
- EventBase<SurveyCreatorModel, ActiveTabChangedEvent>
- Implemented in:
- SurveyCreatorModel
- See also:
- makeNewViewActive
An event that is raised before the active tab is switched. Use this event to allow or cancel the switch.
Parameters:
sender
:SurveyCreatorModel
A Survey Creator instance that raised the event.options.tabName
:string
A tab that is going to become active:"designer"
,"test"
,"theme"
,"editor"
,"logic"
, or"translation"
.options.allow
:boolean
Specifies whether the active tab can be switched. Set this property tofalse
if you want to cancel the switch.
- Type:
- EventBase<SurveyCreatorModel, ActiveTabChangingEvent>
- Implemented in:
- SurveyCreatorModel
- See also:
- makeNewViewActive
An event that is raised before an redo operation.
Parameters:
sender
:SurveyCreatorModel
A Survey Creator instance that raised the event.options.canRedo
:boolean
A Boolean value that you can set tofalse
if you want to prevent the redo operation.
- Type:
- EventBase<SurveyCreatorModel, BeforeRedoEvent>
- Implemented in:
- SurveyCreatorModel
- See also:
- redo * , undo * , onBeforeUndo
An event that is raised before an undo operation.
Parameters:
sender
:SurveyCreatorModel
A Survey Creator instance that raised the event.options.canUndo
:boolean
A Boolean value that you can set tofalse
if you want to prevent the undo operation.
- Type:
- EventBase<SurveyCreatorModel, BeforeUndoEvent>
- Implemented in:
- SurveyCreatorModel
- See also:
- undo * , redo * , onBeforeRedo
An event that is raised when Survey Creator obtains permitted operations for a collection item (a choice option in Choices, a column or row in Columns, etc.). Use this event to prevent users from adding, deleting, or editing a particular collection item.
Parameters:
sender
:SurveyCreatorModel
A Survey Creator instance that raised the event.options.obj
:Base
A survey element (survey, page, panel, question) that contains the collection to which the target item belongs.options.property
:JsonObjectProperty
A property that contains the collection to which the target item belongs.options.propertyName
:string
The property's name:columns
,rows
,choices
,rateValues
, etc.options.collection
:{}
An array of collection items to which the target item belongs (columns
orrows
in matrix questions,choices
in select-based questions, etc.).options.item
:Base
A target collection item.options.allowAdd
:boolean
A Boolean property that you can set tofalse
if you want to prevent the target item from being added to the collection.options.allowDelete
:boolean
A Boolean property that you can set tofalse
if you want to prevent the target item from being deleted.options.allowEdit
:boolean
A Boolean property that you can set tofalse
if you want to prevent the target item from being edited.
- Type:
- EventBase<SurveyCreatorModel, CollectionItemAllowOperationsEvent>
- Implemented in:
- SurveyCreatorModel
- See also:
- onElementAllowOperations
An event that is raised when a condition editor renders a list of questions available for selection. Use this event to modify this list.
Parameters:
sender
:SurveyCreatorModel
A Survey Creator instance that raised the event.options.obj
:Base
A survey element (question, panel, page, or the survey itself) for which the condition editor is displayed.options.propertyName
:string
The name of a property being configured:enableIf
,requiredIf
,visibleIf
, etc.options.editor
:any
A condition editor instance.options.list
:{}
A list of questions available for selection.options.sortOrder
:string
The sort order of questions within the list:"asc"
(default) or"none"
. Set this property to"none"
to disable sorting.
- Type:
- EventBase<SurveyCreatorModel, ConditionGetQuestionListEvent>
- Implemented in:
- SurveyCreatorModel
An event that allows you to create a custom message panel.
Parameters:
sender
:SurveyCreatorModel
A Survey Creator instance that raised the event.options.question
:Question
A question for which the event is raised.options.messageText
:string
A notification message that you want to display. Assign a custom string value to this parameter.options.actionText
:string
A caption text for the action link. Assign a custom string value to this parameter.options.onClick
:() => void
A function that is called when users click the action link. Assign a custom function to this parameter.
A message panel is displayed within a question box on the design surface. It contains a text message and an optional action link. The following image illustrates a built-in message panel that appears when a question sources its choice options from another question or from a web service:
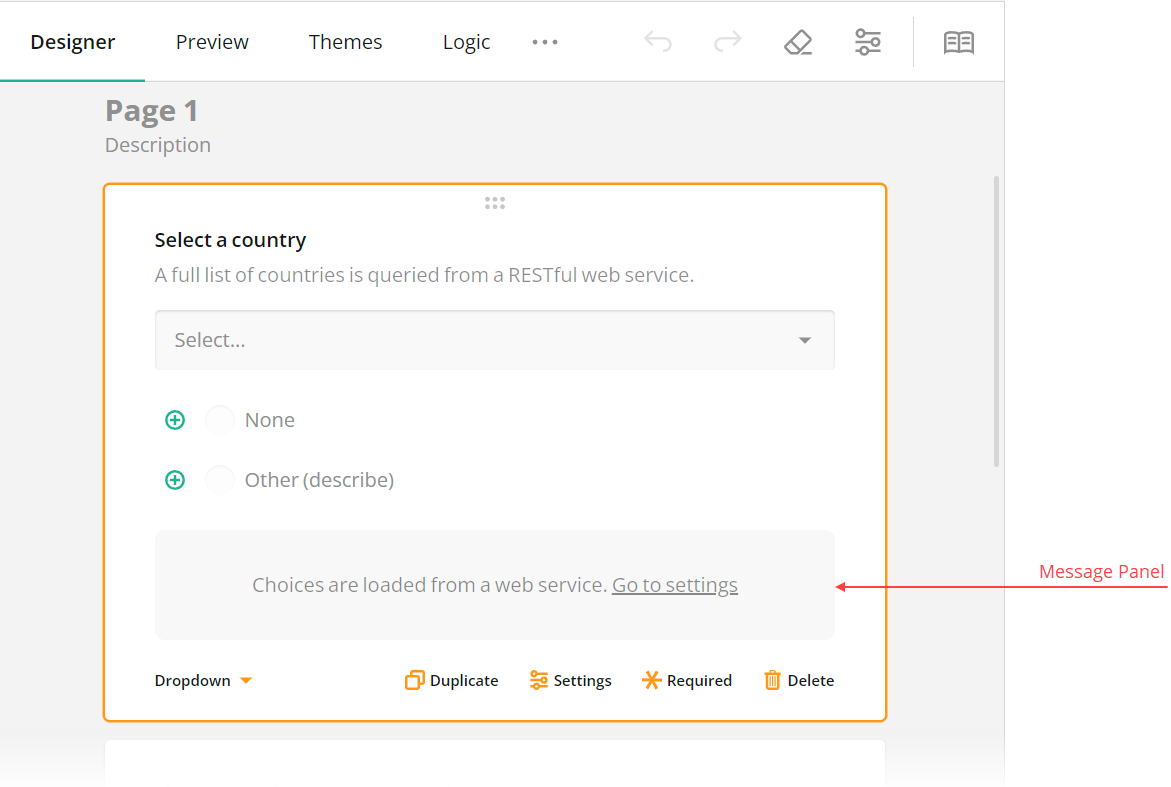
To create a custom message panel, handle the onCreateCustomMessagePanel
event. This event is raised for questions whose isMessagePanelVisible
property set to true
. The following code shows how to enable this property based on a condition. This code implements a custom data source selector for select-based questions (Dropdown, Checkboxes, Radio Button Group). When a survey author selects any data source other than "Custom", the isMessagePanelVisible
property becomes enabled, indicating that the onCreateCustomMessagePanel
event must be raised. A function that handles this event specifies custom message and action link texts and onClick
event handler:
import { Serializer } from "survey-core";
import { SurveyCreatorModel } from "survey-creator-core";
Serializer.addProperty("selectbase", {
name: "choicesDataSource",
displayName: "Data source",
category: "choices",
choices: [
{ text: "Country", value: "country" },
{ text: "Region", value: "region" },
{ text: "City", value: "city" },
{ text: "Custom", value: "custom" }
],
onSetValue: function (obj: any, value: any) {
// Set the custom property value
obj.setPropertyValue("choicesDataSource", value);
// Display the message panel based on a condition
obj.setPropertyValue("isMessagePanelVisible", value !== "custom");
}
});
const creator = new SurveyCreatorModel({});
creator.onCreateCustomMessagePanel.add((_, options) => {
options.messageText = "Choices for this question are loaded from a predefined data source. ";
options.actionText = "Go to settings";
// Focus the "Data source" editor within the Property Grid
options.onClick = () => {
creator.selectElement(options.question, "choicesDataSource");
};
});
- Type:
- EventBase<SurveyCreatorModel, CreateCustomMessagePanelEvent>
- Implemented in:
- SurveyCreatorModel
An event that is raised when Survey Creator obtains adorners for a survey element. Use this event to hide and modify predefined adorners or add a custom adorner.
Parameters:
sender
:SurveyCreatorModel
A Survey Creator instance that raised the event.options.obj
:Base
A survey element (question, panel, or page) whose adorners you can customize.options.items
:{}
An array of adorner actions. You can add, modify, or remove actions from this array.
- Type:
- EventBase<SurveyCreatorModel, ElementGetActionsEvent>
- Implemented in:
- SurveyCreatorModel
- See also:
- onElementAllowOperations * , onGetPageActions
This event is obsolete. Use the onSurveyInstanceCreated
event instead.
Parameters:
sender
:SurveyCreatorModel
A Survey Creator instance that raised the event.options.survey
:SurveyModel
A survey to be displayed in the Designer tab.
- Type:
- EventBase<SurveyCreatorModel, DesignerSurveyCreatedEvent>
- Implemented in:
- SurveyCreatorModel
An event that is raised when users drag and drop survey elements within the design surface. Use this event to control drag and drop operations.
Parameters:
sender
:SurveyCreatorModel
A Survey Creator instance that raised the event.options.draggedElement
:IElement
A survey element being dragged.options.fromElement
:IPanel
A survey element from whichdraggedElement
is being dragged. This parameter isnull
ifdraggedElement
is being dragged from the Toolbox.options.toElement
:IElement
A survey element to whichdraggedElement
is being dragged.options.insertBefore
:IElement
A survey element before whichdraggedElement
will be placed. This parameter isnull
if the parent container (page or panel) has no elements or ifdraggedElement
will be placed below all other elements within the container.options.insertAfter
:IElement
A survey element after whichdraggedElement
will be placed. This parameter isnull
if the parent container (page or panel) has no elements or ifdraggedElement
will be placed above all other elements within the container.options.parent
:ISurveyElement
A parent container (page or panel) within whichdraggedElement
will be placed.options.survey
:SurveyModel
A survey within which the drag and drop operation occured.options.allowDropNextToAnother
:boolean
A Boolean property that you can set tofalse
if you want to disallow placingdraggedElement
on the same line with any other survey element.options.allow
:boolean
A Boolean property that you can set tofalse
if you want to cancel the drag and drop operation.
- Type:
- EventBase<SurveyCreatorModel, DragDropAllowEvent>
- Implemented in:
- SurveyCreatorModel
- See also:
- onDragStart * , onDragEnd
An event that is raised when users finish dragging a survey element within the design surface.
Parameters:
sender
:SurveyCreatorModel
A Survey Creator instance that raised the event.options.draggedElement
:IElement
A survey element being dragged.options.fromElement
:IElement
A survey element from whichdraggedElement
is being dragged. This parameter isnull
ifdraggedElement
is being dragged from the Toolbox.options.toElement
:IElement
A survey element to whichdraggedElement
is being dragged.
- Type:
- EventBase<SurveyCreatorModel, DragStartEndEvent>
- Implemented in:
- SurveyCreatorModel
- See also:
- onDragStart * , onDragDropAllow
An event that is raised when users start to drag a survey element within the design surface.
Parameters:
sender
:SurveyCreatorModel
A Survey Creator instance that raised the event.options.draggedElement
:IElement
A survey element being dragged.options.fromElement
:IElement
A survey element from whichdraggedElement
is being dragged. This parameter isnull
ifdraggedElement
is being dragged from the Toolbox.options.toElement
:IElement
A survey element to whichdraggedElement
is being dragged.
- Type:
- EventBase<SurveyCreatorModel, DragStartEndEvent>
- Implemented in:
- SurveyCreatorModel
- See also:
- onDragEnd * , onDragDropAllow
An event that is raised when Survey Creator obtains permitted operations for a survey element. Use this event to disable user interactions with a question, panel, or page on the design surface.
Parameters:
sender
:SurveyCreatorModel
A Survey Creator instance that raised the event.options.obj
:Base
A survey element (question or panel) for which you can disable user interactions.options.allowChangeRequired
:boolean
Allows users to mark the survey element as required.options.allowChangeType
:boolean
Allows users to change the survey element type.options.allowChangeInputType
:boolean
Allows users to change theinputType
property of Single-Line Input questions.options.allowCopy
:boolean
Allows users to duplicate the survey element.options.allowDelete
:boolean
Allows users to delete the survey element.options.allowDragging
:boolean
Allows users to drag and drop the survey element.options.allowEdit
:boolean
Allows users to edit survey element properties on the design surface. If you disable this property, users can edit the properties only in the Property Grid.options.allowShowSettings
:boolean
Controls the visibility of the Settings button that allows users to open the Property Grid for survey element configuration. Set this property totrue
orfalse
to display or hide the Settings button at all times. The default valueundefined
displays the Settings button only when Survey Creator has small width.
To disable a user interaction, set the correponding allow...
property to false
.
- Type:
- EventBase<SurveyCreatorModel, ElementAllowOperationsEvent>
- Implemented in:
- SurveyCreatorModel
- See also:
- onCollectionItemAllowOperations
An event that is raised before a survey element (a question, panel, or page) is deleted.
Parameters:
sender
:SurveyCreatorModel
A Survey Creator instance that raised the event.options.element
:Base
A survey element to be deleted.options.elementType
:string
The element type:"question"
,"panel"
, or"page"
.options.allowing
:boolean
A Boolean property that you can set tofalse
if you want to cancel element deletion.
- Type:
- EventBase<SurveyCreatorModel, ElementDeletingEvent>
- Implemented in:
- SurveyCreatorModel
- See also:
- deleteElement
An event that is raised when users finish editing collection items (choices, rows, columns) in a pop-up window.
Survey authors can specify collection items using a table UI in Property Grid (see the onItemValueAdded
event) or a multi-line text editor in a pop-up window. Each line in the editor specifies the value and display text of one collection item in the following format: value|text
. Use the onFastEntryFinished
event to process the entered text lines as required.
Parameters:
sender
:SurveyCreatorModel
A Survey Creator instance that raised the event.options.lines
:{}
An array of entered text lines.options.items
:{}
An array of collection items that were created based on the entered text lines. Overwrite an item'svalue
ortext
property if you want to change the value or display text of this item.
- Type:
- EventBase<SurveyCreatorModel, FastEntryItemsEvent>
- Implemented in:
- SurveyCreatorModel
An event that is raised when Survey Creator populates a condition editor with operators. Use this event to hide individual condition operators.
Parameters:
sender
:SurveyCreatorModel
A Survey Creator instance that raised the event.options.questionName
:string
The name of a question for which conditions are displayed.options.operator
:string
A condition operator for which the event is raised:"empty"
,"notempty"
,"equal"
,"notequal"
,"contains"
,"notcontains"
,"anyof"
,"allof"
,"greater"
,"less"
,"greaterorequal"
, or"lessorequal"
.options.show
:boolean
A Boolean property that you can set tofalse
if you want to hide the condition operator.
- Type:
- EventBase<SurveyCreatorModel, GetConditionOperatorEvent>
- Implemented in:
- SurveyCreatorModel
An event that is raised when Survey Creator obtains a survey element name to display it in the UI.
Handle this event to replace survey element names in the UI with custom display texts. If you only want to replace the names with survey element titles, enable the showObjectTitles
property instead of handling this event.
Parameters:
sender
:SurveyCreatorModel
A Survey Creator instance that raised the event.options.obj
:Base
A survey element (survey, page, question, or panel) whose name has been requested.options.area
:string
A Survey Creator UI element that requests the display name. Contains one of the following values:"page-selector"
- Page selector on the design surface."condition-editor"
- Condition pop-up window or drop-down menus that allow users to select questions in the Logic tab."logic-tab:question-filter"
- Question filter in the Logic tab."logic-tab:question-selector"
- Question selector on editing actions in the Logic tab."preview-tab:page-list"
- Page list in the Preview tab."preview-tab:selected-page"
- Selected page name in the Preview tab."property-grid:property-editor"
- Property editors in the Property Grid."property-grid-header:element-list"
- Survey element list in the header of the Property Grid."property-grid-header:selected-element"
- Selected survey element in the header of the Property Grid."translation-tab"
- Translation tab.
options.displayName
:string
A survey element's display text. Modify this property to set a custom display text for the survey element.
- Type:
- EventBase<SurveyCreatorModel, ElementGetDisplayNameEvent>
- Implemented in:
- SurveyCreatorModel
An event that is raised when Survey Creator renders action buttons under each page on the design surface. Use this event to add, remove, or modify the buttons.
Parameters:
sender
:SurveyCreatorModel
A Survey Creator instance that raised the event.options.actions
:{}
An array of actions. You can add, modify, or remove actions from this array.options.page
:PageModel
A page for which the event is raised.options.addNewQuestion
:(type: string) => void
Adds a new question of a specifiedtype
to the page.
- Type:
- EventBase<SurveyCreatorModel, PageGetFooterActionsEvent>
- Implemented in:
- SurveyCreatorModel
- See also:
- onDefineElementMenuItems
An event that is raised when Survey Creator sets the read-only status for a survey element property. Use this event to change the read-only status for individual properties.
Parameters:
sender
:SurveyCreatorModel
A Survey Creator instance that raised the event.options.property
:JsonObjectProperty
A property whose read-only status you can change.options.parentProperty
:JsonObjectProperty
A property that nestsoptions.property
(choices
for an item value,columns
for a matrix column,rows
for a matrix row etc.).options.parentProperty
has a value only for nested properties.options.obj
:Base
A survey element (question, panel, page, or the survey itself) for which you can change the read-only status.options.parentObj
:Base
A survey element that containsoptions.parentProperty
.options.parentObj
has a value only for nested properties.options.readOnly
:boolean
A Boolean value that specifies the property's read-only status.
- Type:
- EventBase<SurveyCreatorModel, PropertyGetReadOnlyEvent>
- Implemented in:
- SurveyCreatorModel
An event that is raised after a user has edited a text value on the design surface. This value may include HTML markup. You can handle the onHtmlToMarkdown
event to convert the HTML markup to Markdown.
Parameters:
sender
:SurveyCreatorModel
A Survey Creator instance that raised the event.options.element
:Base
The instance of a survey element (survey, page, panel, question) that the user configures.options.name
:string
The name of a property whose value has been edited.options.html
:string
HTML content. Pass this field's value to an HTML-to-Markdown converter.options.text
:string
A text string that may contain Markdown. Assign the result of the HTML-to-Markdown conversion to this field.
- Type:
- EventBase<SurveyCreatorModel, HtmlToMarkdownEvent>
- Implemented in:
- SurveyCreatorModel
An event that is raised when users add a new collection item (a choice option to Choices, a column or row to Columns, etc.). Use this event to modify this item.
Parameters:
sender
:SurveyCreatorModel
A Survey Creator instance that raised the event.options.obj
:Base
A survey element (survey, page, panel, question) that contains the collection to which the target item belongs.options.propertyName
:string
The property's name:columns
,rows
,choices
,rateValues
, etc.options.newItem
:ItemValue
A new collection item. Overwrite itsvalue
ortext
property if you want to change the item's value or display text.options.itemValues
:{}
An array of collection items to which the target item belongs (columns
orrows
in matrix questions,choices
in select-based questions, etc.).
This event is not raised when users add a new column to a Multi-Select Matrix or Dynamic Matrix. For these cases, handle the
onMatrixColumnAdded
event instead.
- Type:
- EventBase<SurveyCreatorModel, CollectionItemAddedEvent>
- Implemented in:
- SurveyCreatorModel
- See also:
- onFastEntryFinished * , onCollectionItemAllowOperations
An event that is raised when an ItemValue
property is changed.
Parameters:
sender
:this
A SurveyJS object whose property contains an array ofItemValue
objects.options.obj
:ItemValue
AnItemValue
object.options.propertyName
:string
The name of the property to which an array ofItemValue
objects is assigned (for example,"choices"
or"rows"
).options.name
:"text"
|"value"
The name of the changed property.options.newValue
:any
A new value for the property.
- Type:
- Event<(sender: Base, options: any) => any, Base, any>
- Implemented in:
- Base
An event that is raised when the Logic tab constructs a user-friendly display text for a logic rule. Use this event to modify this display text.
Parameters:
sender
:SurveyCreatorModel
A Survey Creator instance that raised the event.options.expression
:string
A logical expression associated with the logic rule.options.expressionText
:string
The same expression in a user-friendly format.options.logicItem
:SurveyLogicItem
An object that describes the logic rule. Contains an array of actions and other properties.options.text
:string
A user-friendly display text for the logic rule. Modify this parameter if you want to override the display text.
- Type:
- EventBase<SurveyCreatorModel, LogicRuleGetDisplayTextEvent>
- Implemented in:
- SurveyCreatorModel
An event that allows you to integrate a machine translation service, such as Google Translate or Microsoft Translator, into Survey Creator.
Parameters:
sender
:SurveyCreatorModel
A Survey Creator instance that raised the event.options.fromLocale
:string
A locale from which you want to translate strings. Contains a locale code ("en"
,"de"
, etc.).options.toLocale
:string
A locale to which you want to translate strings. Contains a locale code ("en"
,"de"
, etc.).options.strings
:any
Strings to translate.options.callback
:(strings: any) => void
A callback function that accepts translated strings. If the translation failed, pass an empty array or call this function without arguments.
Within the event handler, you need to pass translation strings and locale information to the translation service API. The service should return an array of translated strings that you need to pass to the options.callback
function. The following code shows how to integrate the Microsoft Translator service into Survey Creator:
import { SurveyCreatorModel } from "survey-creator-core";
const creatorOptions = { ... };
const creator = new SurveyCreatorModel(creatorOptions);
const apiKey = "<your-microsoft-translator-api-key>";
const resourceRegion = "<your-azure-region>";
const endpoint = "https://api.cognitive.microsofttranslator.com/";
creator.onMachineTranslate.add((_, options) => {
// Prepare strings for Microsoft Translator as an array of objects: [{ Text: "text to translate" }]
const data = [];
options.strings.forEach(str => { data.push({ Text: str }); });
// Include required locales in the URL
const params = "api-version=3.0&from=" + options.fromLocale + "&to=" + options.toLocale;
const url = endpoint + "/translate?" + params;
fetch(url, {
method: "POST",
headers: {
"Content-Type": "application/json",
"Ocp-Apim-Subscription-Key": apiKey,
"Ocp-Apim-Subscription-Region": resourceRegion,
"X-ClientTraceId": crypto.randomUUID()
},
body: JSON.stringify(data)
}).then(response => response.json())
.then(data => {
// Convert data received from Microsoft Translator to a flat array
const translatedStrings = [];
for (let i = 0; i < data.length; i++) {
translatedStrings.push(data[i].translations[0].text);
}
// Pass translated strings to Survey Creator
options.callback(translatedStrings);
}).catch(error => {
// If translation was unsuccessful:
options.callback();
alert("Could not translate strings to the " + options.toLocale + " locale");
});
});
Survey Creator does not include a machine translation service out of the box. Our component only provides a UI for calling the service API.
- Type:
- EventBase<SurveyCreatorModel, MachineTranslateEvent>
- Implemented in:
- SurveyCreatorModel
An event that is raised when users add a new column to a Multi-Select Matrix or Dynamic Matrix. Use this event to modify this column.
Parameters:
sender
:SurveyCreatorModel
A Survey Creator instance that raised the event.options.matrix
:Question
A Multi-Select or Dynamic Matrix to which a new column is being added.options.newColumn
:MatrixDropdownColumn
A new matrix column. Edit its properties if you want to modify this column.options.columns
:{}
An array of matrix columns.
This event is not raised when users add a new column to a Single-Select Matrix. For this case, handle the
onItemValueAdded
event instead.
- Type:
- EventBase<SurveyCreatorModel, MatrixColumnAddedEvent>
- Implemented in:
- SurveyCreatorModel
- See also:
- onCollectionItemAllowOperations
An event that is raised when users modify survey or theme settings.
Parameters:
sender
:SurveyCreatorModel
A Survey Creator instance that raised the event.options.type
:string
A value that indicates the modification:"ADDED_FROM_TOOLBOX"
, "ADDED_FROM_PAGEBUTTON","PAGE_ADDED"
,"QUESTION_CONVERTED"
,"ELEMENT_COPIED"
,"PROPERTY_CHANGED"
,"ELEMENT_REORDERED"
,"OBJECT_DELETED"
,"JSON_EDITOR"
Depending on the options.type
value, the options
object contains parameters listed below:
options.type
: "ADDED_FROM_TOOLBOX"
| "ADDED_FROM_PAGEBUTTON"
| "ELEMENT_COPIED"
options.question
- An added or copied survey element.
options.type
: "PAGE_ADDED"
options.newValue
- An added page.
options.type
: "QUESTION_CONVERTED"
options.className
- The name of a class to which a question has been converted.options.oldValue
- An object of a previous class.options.newValue
- An object of a class specified byoptions.className
.
options.type
: "PROPERTY_CHANGED"
options.name
- The name of the changed property.options.target
- An object that contains the changed property.options.oldValue
- A previous value of the changed property.options.newValue
- A new value of the changed property.
options.type
: "ELEMENT_REORDERED"
options.arrayName
- The name of the changed array.options.parent
- An object that contains the changed array.options.element
- A reordered element.options.indexFrom
- A previous index.options.indexTo
- A current index.
options.type
: "OBJECT_DELETED"
options.target
- A deleted object.
- Type:
- EventBase<SurveyCreatorModel, ModifiedEvent>
- Implemented in:
- SurveyCreatorModel
- See also:
- state
An event that is raised when Survey Creator displays a toast notification. Use this event to implement custom toast notification.
Parameters:
sender
:SurveyCreatorModel
A Survey Creator instance that raised the event.options.message
:string
A message to display.options.type
:string
A notification type:"info"
or"error"
.
- Type:
- EventBase<SurveyCreatorModel, NotifyEvent>
- Implemented in:
- SurveyCreatorModel
- See also:
- notify
An event that is raised when Survey Creator opens a dialog window for users to select files.
Parameters:
sender
:SurveyCreatorModel
A Survey Creator instance that raised the event.options.input
:HTMLInputElement
A file input HTML element.options.element
:Base | ITheme
A survey element (question, panel, page, or survey) or a theme JSON schema for which this event is raised.options.elementType
:any
The type of the element passed as theoptions.element
parameter.
Possible values:"theme"
,"header"
, or any value returned from thegetType()
method.options.propertyName
:any
The name of the survey element property or theme property for which files are being selected.options.item
:ItemValue
A choice item for which the event is raised. This parameter has a value only when the dialog window is opened to select images for an Image Picker question.options.callback
:(files: any) => void
A callback function to which you should pass selected files.
- Type:
- EventBase<SurveyCreatorModel, OpenFileChooserEvent>
- Implemented in:
- SurveyCreatorModel
- See also:
- onUploadFile * , uploadFiles
An event that is raised when a new page is added to the survey. Use this event to customize the page.
Parameters:
sender
:SurveyCreatorModel
A Survey Creator instance that raised the event.options.page
:PageModel
The page users added.
Customize Survey Elements on Creation
- Type:
- EventBase<SurveyCreatorModel, PageAddedEvent>
- Implemented in:
- SurveyCreatorModel
An event that is raised before a new page is added to the survey. Handle this event if you do not want to add the page.
Parameters:
sender
:SurveyCreatorModel
A Survey Creator instance that raised the event.options.page
:PageModel
A page to be added.options.allow
:boolean
Set this property tofalse
if you do not want to add the page.
- Type:
- EventBase<SurveyCreatorModel, PageAddingEvent>
- Implemented in:
- SurveyCreatorModel
An event that is raised when users add a Panel element to the survey. Use this event to customize the panel.
Parameters:
sender
:SurveyCreatorModel
A Survey Creator instance that raised the event.options.panel
:PanelModel
The panel users added.options.page
:PageModel
A page to which the panel was added.options.reason
:string
A value that indicates how the panel was added: dragged from the Toolbox ("DROPPED_FROM_TOOLBOX"
), created using the Add Question button ("ADDED_FROM_PAGEBUTTON"
), or duplicated ("ELEMENT_COPIED"
).
Customize Survey Elements on Creation
- Type:
- EventBase<SurveyCreatorModel, PanelAddedEvent>
- Implemented in:
- SurveyCreatorModel
This event is obsolete. Use the onSurveyInstanceCreated
event instead.
Parameters:
sender
:SurveyCreatorModel
A Survey Creator instance that raised the event.options.survey
:SurveyModel
A survey to be displayed in the Preview tab.
- Type:
- EventBase<SurveyCreatorModel, PreviewSurveyCreatedEvent>
- Implemented in:
- SurveyCreatorModel
An event that is raised when a property of this SurveyJS object has changed.
Parameters:
sender
:this
A SurveyJS object whose property has changed.options.name
:string
The name of the changed property.options.newValue
:any
A new value for the property.options.oldValue
:any
An old value of the property. If the property is an array,oldValue
contains the same array asnewValue
does.
If you need to add and remove property change event handlers dynamically, use the registerPropertyChangedHandlers
and unregisterPropertyChangedHandlers
methods instead.
- Type:
- EventBase<Base, any>
- Implemented in:
- Base
An event that is raised when a property editor is created in the Property Grid. Use this event to modify the property editor or add event handlers to it.
Parameters:
sender
:SurveyCreatorModel
A Survey Creator instance that raised the event.options.editor
:Question
A property editor. It is an object of theQuestion
type because the Property Grid is built upon a regular survey.options.property
:JsonObjectProperty
A property that corresponds to the created property editor.options.obj
:Base
A survey element being edited in the Property Grid.
- Type:
- EventBase<SurveyCreatorModel, PropertyEditorCreatedEvent>
- Implemented in:
- SurveyCreatorModel
- See also:
- onSetPropertyEditorOptions * , onSurveyInstanceCreated
An event that is raised when title actions are added to a property editor. Title actions are most often used to reveal hints for properties configured by users. Handle this event you want to add, remove, or modify the title actions.
Parameters:
sender
:SurveyCreatorModel
A Survey Creator instance that raised the event.options.obj
:Base
A survey element being edited in the Property Grid.options.editor
:Question
A property editor that contains the title actions. It is an object of theQuestion
type because the Property Grid is built upon a regular survey.options.property
:JsonObjectProperty
A property that corresponds to the property editor.options.titleActions
:{}
A list of title actions.
- Type:
- EventBase<SurveyCreatorModel, PropertyEditorUpdateTitleActionsEvent>
- Implemented in:
- SurveyCreatorModel
An event that is raised before Survey Creator displays a pop-up window called from the Property Grid. This window allows users to edit choices, conditions, etc. Use this event to customize the pop-up window, for example, add custom action buttons.
Parameters:
sender
:SurveyCreatorModel
A Survey Creator instance that raised the event.options.obj
:Base
The instance of a survey element (question or panel) that users are configuring in the Property Grid.options.property
:JsonObjectProperty
A property being edited.options.editor
:Question
A property editor. It is an object of theQuestion
type because the Property Grid is built upon a regular survey.options.popupEditor
:any
An editor inside the pop-up window.options.popupModel
:PopupBaseViewModel
A pop-up window model. Useoptions.popupModel.footerToolbar
to access the actions at the bottom of the window.
- Type:
- EventBase<SurveyCreatorModel, PropertyGridShowPopupEvent>
- Implemented in:
- SurveyCreatorModel
This event is obsolete. Use the onSurveyInstanceCreated
event instead.
Parameters:
sender
:SurveyCreatorModel
A Survey Creator instance that raised the event.options.obj
:Base
A survey element being edited in the Property Grid.options.survey
:SurveyModel
A survey that represents the Property Grid. Use theSurveyModel
API to modify the survey.
- Type:
- EventBase<SurveyCreatorModel, PropertyGridSurveyCreatedEvent>
- Implemented in:
- SurveyCreatorModel
An event that is raised when Survey Creator validates a modified value of a survey element property. Use this event to display a custom error message when the value is incorrect.
Parameters:
sender
:SurveyCreatorModel
A Survey Creator instance that raised the event.options.obj
:Base
A survey element (survey, page, panel, question) whose property is being validated.options.propertyName
:string
The name of a property being validated.options.value
:any
The property value.options.error
:string
An error message you want to display. Ifoptions.value
is valid, this parameter contains an empty string.
- Type:
- EventBase<SurveyCreatorModel, PropertyDisplayCustomErrorEvent>
- Implemented in:
- SurveyCreatorModel
An event that is raised each time a user modifies a survey element property. Use this event to validate or correct a property value while the user changes it.
Parameters:
sender
:SurveyCreatorModel
A Survey Creator instance that raised the event.options.obj
:Base
A survey element (question, panel, page, or the survey itself) whose property is being edited.options.propertyName
:string
The name of a property being modified.options.value
:any
An old property value.options.newValue
:any
A new property value. Modify this parameter if you want to override the property value.
- Type:
- EventBase<SurveyCreatorModel, PropertyValueChangingEvent>
- Implemented in:
- SurveyCreatorModel
An event that is raised when users add a question to the survey. Use this event to customize the question.
Parameters:
sender
:SurveyCreatorModel
A Survey Creator instance that raised the event.options.question
:Question
The question users added.options.page
:PageModel
A page to which the question was added.options.reason
:string
A value that indicates how the question was added: dragged from the Toolbox ("DROPPED_FROM_TOOLBOX"
), created using the Add Question button ("ADDED_FROM_PAGEBUTTON"
), duplicated ("ELEMENT_COPIED"
), or converted from another question type ("ELEMENT_CONVERTED"
).
Customize Survey Elements on Creation
- Type:
- EventBase<SurveyCreatorModel, QuestionAddedEvent>
- Implemented in:
- SurveyCreatorModel
An event that is raised when a question's type is being changed.
Parameters:
sender
:SurveyCreatorModel
A Survey Creator instance that raised the event.options.sourceQuestion
:Question
A question of the previous type.options.targetType
:string
A target type.options.json
:any
A JSON object produced by the previous question. You can modify this object to decide which properties should be copied to a new question. Set this parameter toundefined
if you want to create the new question with a default JSON object.
- Type:
- EventBase<SurveyCreatorModel, QuestionConvertingEvent>
- Implemented in:
- SurveyCreatorModel
An event that is raised after a survey element (a question, panel, page, or the survey itself) is focused.
Parameters:
sender
:SurveyCreatorModel
A Survey Creator instance that raised the event.options.newSelectedElement
:Base
The focused element.
- Type:
- EventBase<SurveyCreatorModel, ElementFocusedEvent>
- Implemented in:
- SurveyCreatorModel
- See also:
- onSelectedElementChanging
An event that is raised before a survey element (question, panel, page, or the survey itself) is focused. Use this event to move focus to a different survey element.
Parameters:
sender
:SurveyCreatorModel
A Survey Creator instance that raised the event.options.newSelectedElement
:Base
An element that is going to be focused.
- Type:
- EventBase<SurveyCreatorModel, ElementFocusingEvent>
- Implemented in:
- SurveyCreatorModel
- See also:
- onSelectedElementChanged * , selectedElement
An event that is raised when a table property editor is created in the Property Grid. Use this event to configure the table property editor.
Parameters:
sender
:SurveyCreatorModel
A Survey Creator instance that raised the event.options.obj
:Base
A survey element (survey, page, panel, question) for which the table property editor is created.options.propertyName
:string
The name of the property with which the editor is associated:"columns"
,"rows"
,"choices"
, etc.options.editorOptions
:TablePropertyEditorOptions
An obejct with table property editor settings that you can modify.
- Type:
- EventBase<SurveyCreatorModel, ConfigureTablePropertyEditorEvent>
- Implemented in:
- SurveyCreatorModel
- See also:
- onPropertyEditorCreated
An event that is raised when Survey Creator adds properties to a survey element selected on the design surface. Handle this event if you cancel the addition of certain properties and thus hide them from the Property Grid.
Parameters:
sender
:SurveyCreatorModel
A Survey Creator instance that raised the event.options.property
:JsonObjectProperty
A property being added.options.parentProperty
:JsonObjectProperty
A property that nestsoptions.property
(choices
for an item value,columns
for a matrix column,rows
for a matrix row etc.).options.parentProperty
has a value only for nested properties.options.obj
:Base
A survey element that containsoptions.property
: page, panel, question, the survey itself, item value (choice option), matrix column, etc.options.parentObj
:Base
A survey element that containsoptions.parentProperty
.options.parentObj
has a value only for nested properties.options.canShow
:boolean
A Boolean property that you can set tofalse
if you do not want to add the property.
- Type:
- EventBase<SurveyCreatorModel, PropertyAddingEvent>
- Implemented in:
- SurveyCreatorModel
An event that is raised when Survey Creator instantiates a survey to display a UI element. Handle this event to customize the UI element by modifying the survey.
Parameters:
sender
:SurveyCreatorModel
A Survey Creator instance that raised the event.options.area
:string
A Survey Creator UI element for which a survey is instantiated. Contains one of the following values:"designer-tab"
- A preview survey in the Designer tab."preview-tab"
- A preview survey in the Preview tab."property-grid"
- A survey that represents the Property Grid."default-value-popup-editor"
- A survey that allows you to specify the default or correct value (for quizzes) in a pop-up window."logic-rule:condition-editor"
- A survey that allows you to configure conditions in a logic rule."logic-rule:action-editor"
- A survey that allows you to configure actions in a logic rule."logic-tab:condition-list"
- A survey that displays the list of logic rules in the Logic tab."theme-tab"
- A preview survey in the Themes tab."theme-tab:property-grid"
- A survey that represents the Property Grid in the Themes tab."translation-tab:language-list"
- A survey that displays the language list in the Translations tab."translation-tab:table"
- A survey that displays the translation table in the Translations tab."translation-tab:table-header"
- A survey that displays the header of the translation table in the Translations tab."translation-tab:table-popup-editor"
- A survey that displays a translation table for an individual language in a pop-up window."table-values-popup-editor"
- A survey that allows you to edit values of a table (Choices, Rows, Columns, etc.) in a pop-up window."matrix-cell-values-popup-editor"
- A survey that allows you to specify cell texts of a Single-Select Matrix in a pop-up window."matrix-cell-question-popup-editor"
- A survey that allows you to configure a question within a cell of a Multi-Select or Dynamic Matrix in a pop-up window.
options.survey
:SurveyModel
A survey that represents the Survey Creator UI element to be displayed. Use theSurveyModel
API to modify the survey.
If you want this event raised at startup, assign a survey JSON schema to the
JSON
property after you add a handler to the event. If the JSON schema should be empty, specify theJSON
property with an empty object.
- Type:
- EventBase<SurveyCreatorModel, SurveyInstanceCreatedEvent>
- Implemented in:
- SurveyCreatorModel
An event that is raised after a survey element property has changed.
Parameters:
sender
:SurveyCreatorModel
A Survey Creator instance that raised the event.options.obj
:Base
A survey element (question, panel, page, or the survey itself) whose property has changed.options.propertyName
:string
The name of the modified property.options.value
:any
A new property value.
- Type:
- EventBase<SurveyCreatorModel, PropertyValueChangedEvent>
- Implemented in:
- SurveyCreatorModel
An event that is raised before a translated string is exported to a CSV file. Use this event to modify the string to be exported.
Parameters:
sender
:SurveyCreatorModel
A Survey Creator instance that raised the event.options.obj
:Base
A survey element (survey, page, panel, question) whose string translations are being exported to CSV.options.locale
:string
The current locale code ("en"
,"de"
, etc.). Contains an empty string if the default locale is used.options.name
:string
A full name of the translated string. It is composed of names of all parent elements, for example:"mySurvey.page1.question2.title"
.options.locString
:LocalizableString
ALocalizableString
instance. Call theoptions.locString.getLocaleText(locale)
method if you need to get a text string for a specific locale.options.text
:string
A text string to be exported. The string is taken from the current locale. You can modify this property to export a different string.
- Type:
- EventBase<SurveyCreatorModel, TranslationExportItemEvent>
- Implemented in:
- SurveyCreatorModel
- See also:
- onTranslationImportItem
An event that is raised after all translated strings are imported from a CSV file.
Parameters:
sender
:SurveyCreatorModel
A Survey Creator instance that raised the event.
- Type:
- EventBase<SurveyCreatorModel, TranslationImportedEvent>
- Implemented in:
- SurveyCreatorModel
- See also:
- onTranslationImportItem * , onTranslationExportItem
An event that is raised before a translated string is imported from a CSV file. Use this event to modify the string to be imported or cancel the import.
Parameters:
sender
:SurveyCreatorModel
A Survey Creator instance that raised the event.options.locale
:string
The current locale code ("en"
,"de"
, etc.). Contains an empty string if the default locale is used.options.name
:string
A full name of the translated string. It is composed of names of all parent elements, for example:"mySurvey.page1.question2.title"
.options.text
:string
A text string to be imported. You can modify this property to import a different string or set this property toundefined
to cancel the import.
- Type:
- EventBase<SurveyCreatorModel, TranslationImportItemEvent>
- Implemented in:
- SurveyCreatorModel
- See also:
- onTranslationExportItem * , onTranslationImported
An event that is raised before a string translation is changed. Use this event to override a new translation value.
Parameters:
sender
:SurveyCreatorModel
A Survey Creator instance that raised the event.options.obj
:Base
A survey element instance (survey, page, panel, question) whose string translation is being changed.options.locale
:string
The current locale code ("en"
,"de"
, etc.). Contains an empty string if the default locale is used.options.locString
:LocalizableString
An object that you can use to manipulate a localization string. Call theoptions.locString.getLocaleText(locale)
method if you need to get a text string for a specific locale.options.newText
:string
A new value for the string translation.
Refer to the following help topics for more information on localization:
- Localization & Globalization in SurveyJS Form Library
- Localization & Globalization in Survey Creator
- Type:
- EventBase<SurveyCreatorModel, TranslationItemChangingEvent>
- Implemented in:
- SurveyCreatorModel
An event that is raised when the Translation tab displays a property for translation. Use this event to control the property visibility.
Parameters:
sender
:SurveyCreatorModel
A Survey Creator instance that raised the event.options.obj
:Base
A survey element (survey, page, panel, question) whose string translations are edited in the Translation tab.options.propertyName
:string
The name of a property being translated.options.visible
:boolean
A Boolean value that specifies the property visibility. Set it tofalse
to hide the property.
- Type:
- EventBase<SurveyCreatorModel, TranslationStringVisibilityEvent>
- Implemented in:
- SurveyCreatorModel
An event that is raised when a user selects a file to upload. Use this event to upload the file to your server.
Parameters:
sender
:SurveyCreatorModel
A Survey Creator instance that raised the event.options.element
:Base | ITheme
A survey element (question, panel, page, or survey) or a theme JSON schema for which this event is raised.options.elementType
:any
The type of the element passed as theoptions.element
parameter.
Possible values:"theme"
,"header"
, or any value returned from thegetType()
method.options.propertyName
:any
The name of the survey element property or theme property for which files are being uploaded.options.files
:{}
Files to upload.options.callback
:(status: string, fileUrl?: string) => void
A callback function that you should call when a file is uploaded successfully or when file upload fails. Pass"success"
or"error"
as thestatus
argument. If the file upload is successful, pass the file's URL as thefileUrl
argument.
- Type:
- EventBase<SurveyCreatorModel, UploadFileEvent>
- Implemented in:
- SurveyCreatorModel
- See also:
- uploadFiles
Specifies how Survey Creator users edit survey pages.
Accepted values:
"standard"
(default)
Questions and panels are divided between pages. Users can scroll the design surface to reach a required page."single"
All questions and panels belong to a single page. Users cannot add or remove pages."bypage"
Questions and panels are divided between pages. Users can use the page navigator to switch to a required page.
- Type:
- "standard" | "single" | "bypage" writable
- Implemented in:
- SurveyCreatorModel
- See also:
- allowModifyPages
Specifies the orientation of the selected device in the Preview tab.
Possible values:
"landscape"
(default)"portrait"
- Type:
- "landscape" | "portrait" readonly
- Implemented in:
- SurveyCreatorModel
Specifies whether to display a table with survey results after completing a survey in the Preview tab.
Default value: true
- Type:
- boolean readonly
- Implemented in:
- SurveyCreatorModel
Enables the read-only mode. If you set this property to true
, users cannot change the initial survey configuration.
Default value: false
- Type:
- boolean writable
- Implemented in:
- SurveyCreatorModel
Repeats the last undone action if possible.
- Type:
- () => void
- Implemented in:
- SurveyCreatorModel
- See also:
- undo * , onBeforeRedo
Refreshes the Designer tab.
refreshDesigner()
is useful if the Designer tab UI depends on an external variable. Call this method each time this variable changes to update the UI.
- Type:
- () => void
- Implemented in:
- SurveyCreatorModel
Registers a single value change handler for one or multiple properties.
The registerPropertyChangedHandlers
and unregisterPropertyChangedHandlers
methods allow you to manage property change event handlers dynamically. If you only need to attach an event handler without removing it afterwards, you can use the onPropertyChanged
event instead.
- Type:
- (propertyNames: any, handler: any, key?: string) => void
- Parameters:
-
propertyNames, type: any ,
An array of one or multiple property names.
handler, type: any ,A function to call when one of the listed properties change. Accepts a new property value as an argument.
key, type: string ,(Optional) A key that identifies the current registration. If a function for one of the properties is already registered with the same key, the function will be overwritten. You can also use the key to subsequently unregister handlers.
- Implemented in:
- Base
- See also:
- unregisterPropertyChangedHandlers
Calls the saveSurveyFunc
and saveThemeFunc
functions to save the survey and theme JSON schemas.
- Type:
- () => void
- Implemented in:
- SurveyCreatorModel
- See also:
- saveSurvey * , saveTheme
Calls the saveSurveyFunc
function to save the survey JSON schema.
- Type:
- () => void
- Implemented in:
- SurveyCreatorModel
- See also:
- saveTheme * , save
A function that is called each time users click the Save button or auto-save is triggered to save a survey JSON schema.
For more information, refer to the Save and Load Survey Model Schemas help topic for your framework: Angular | Vue | React | Knockout / jQuery.
- Type:
- any writable
- Implemented in:
- SurveyCreatorModel
- See also:
- saveThemeFunc
Calls the saveThemeFunc
function to save the theme JSON schema.
- Type:
- () => void
- Implemented in:
- SurveyCreatorModel
- See also:
- saveSurvey * , save
A function that is called each time users click the Save button or auto-save is triggered to save a theme JSON object.
For more information, refer to the Save and Load Custom Themes help topic.
- Type:
- any writable
- Implemented in:
- SurveyCreatorModel
- See also:
- showThemeTab * , themeEditor * , saveSurveyFunc
Gets or sets the focused survey element: a question, panel, page, or the survey itself.
- Type:
- Base writable
- Implemented in:
- SurveyCreatorModel
- See also:
- onSelectedElementChanging * , onSelectedElementChanged
Assigns a new value to a specified property.
- Type:
- (name: string, val: any) => void
- Parameters:
-
name, type: string ,
A property name.
val, type: any ,A new value for the property.
- Implemented in:
- Base
Specifies whether the Preview tab displays a language selector.
Accepted values:
"auto"
(default)
Display the language selector only if the survey is translated into more than one language.true
Always display the language selector regardless of how many languages the survey uses.false
Never display the language selector."all"
Always display the language selector with all supported languages.
- Type:
- string | boolean writable
- Implemented in:
- SurveyCreatorModel
Specifies whether to display the Designer tab.
Default value: true
- Type:
- boolean readonly
- Implemented in:
- SurveyCreatorModel
- See also:
- activeTab * , onSurveyInstanceCreated
Specifies whether to show an error message if a survey is not saved in a database.
Default value: true
- Type:
- boolean readonly
- Implemented in:
- SurveyCreatorModel
Specifies whether the Preview tab displays a toggle that allows users to show or hide invisible survey elements.
Default value: true
- Type:
- boolean writable
- Implemented in:
- SurveyCreatorModel
Specifies whether to display the JSON Editor tab.
Default value: true
- Type:
- boolean readonly
- Implemented in:
- SurveyCreatorModel
- See also:
- activeTab
Specifies whether to display the Logic tab.
Default value: false
- Type:
- boolean readonly
- Implemented in:
- SurveyCreatorModel
- See also:
- activeTab
Specifies whether drop-down menus and other UI elements display survey, page, and question titles instead of their names.
Default value: false
- Type:
- boolean readonly
- Implemented in:
- SurveyCreatorModel
- See also:
- onGetObjectDisplayName
Specifies whether to show a page selector at the bottom of the Preview tab.
Default value: true
- Type:
- boolean writable
- Implemented in:
- SurveyCreatorModel
Specifies whether to display the Preview tab.
Default value: true
- Type:
- boolean writable
- Implemented in:
- SurveyCreatorModel
- See also:
- activeTab * , onSurveyInstanceCreated
Specifies whether to display a button that saves the survey or theme (executes the saveSurveyFunc
or saveThemeFunc
function).
Default value: false
- Type:
- boolean readonly
- Implemented in:
- SurveyCreatorModel
- See also:
- isAutoSave * , syncSaveButtons
Specifies whether to show the sidebar that displays the Property Grid.
Default value: true
- Type:
- boolean writable
- Implemented in:
- SurveyCreatorModel
- See also:
- sidebarLocation
Specifies whether the Preview tab displays a Device button that allows users to preview the survey on different device types.
Default value: true
- Type:
- boolean writable
- Implemented in:
- SurveyCreatorModel
Specifies whether users can see and edit the survey header and related survey properties.
Default value: true
- Type:
- boolean writable
- Implemented in:
- SurveyCreatorModel
Specifies whether to display the Themes tab.
Default value: false
Use the themeEditor
object to manage UI themes available in the Themes tab.
- Type:
- boolean readonly
- Implemented in:
- SurveyCreatorModel
- See also:
- activeTab * , saveThemeFunc
Specifies whether to display question titles instead of names when users edit logical expressions.
Default value: false
- Type:
- boolean readonly
- Implemented in:
- SurveyCreatorModel
- See also:
- showObjectTitles * , onGetObjectDisplayName
Specifies whether to display the Translation tab.
Default value: false
- Type:
- boolean readonly
- Implemented in:
- SurveyCreatorModel
- See also:
- activeTab
Specifies the position of the sidebar that displays the Property Grid. Applies only when showSidebar
is true
.
Possible values:
"right"
(default) - Displays the sidebar on the right side of the design surface."left"
- Displays the sidebar on the left side of the design surface.
- Type:
- "left" | "right" readonly
- Implemented in:
- SurveyCreatorModel
- See also:
- toolboxLocation
Indicates the state of Survey Creator.
Possible values:
""
- Survey Creator doesn't have unsaved changes."modified"
- Survey Creator has unsaved changes."saving"
- Changes are being saved."saved"
- Changes are successfully saved.
- Type:
- string readonly
- Implemented in:
- SurveyCreatorModel
- See also:
- onModified
A survey being configured in the Designer tab.
- Type:
- SurveyModel readonly
- Implemented in:
- SurveyCreatorModel
- See also:
- onSurveyInstanceCreated
Specifies whether to synchronize Save buttons in the Designer and Themes tabs.
Default value: false
When this property is disabled, the Save button in the Designer tab saves only the survey JSON schema, while the Save button in the Themes tab saves only the theme JSON schema. If you enable this property, both buttons will save both JSON schemas.
- Type:
- boolean readonly
- Implemented in:
- SurveyCreatorModel
- See also:
- saveSurveyFunc * , saveThemeFunc * , save
A survey JSON schema as a string.
This property allows you to get or set the JSON schema of a survey being configured. Alternatively, you can use the JSON
property.
- Type:
- string writable
- Implemented in:
- SurveyCreatorModel
Gets or sets a theme for the survey being configured.
- Type:
- ITheme writable
- Implemented in:
- SurveyCreatorModel
- See also:
- showThemeTab * , themeEditor * , saveThemeFunc
An object that enables you to manage UI themes. Refer to the following API section for information on available properties, methods, and events: ThemeTabPlugin
.
- Type:
- ThemeTabPlugin readonly
- Implemented in:
- SurveyCreatorModel
- See also:
- showThemeTab * , saveThemeFunc
A UI theme used to display the survey in the Preview tab.
Accepted values: "modern"
, "default"
, "defaultV2"
Default value: "defaultV2"
- Type:
- string readonly
- Implemented in:
- SurveyCreatorModel
- See also:
- allowChangeThemeInPreview
Provides access to the Toolbox API.
- Type:
- QuestionToolbox readonly
- Implemented in:
- SurveyCreatorModel
Specifies the Toolbox location.
Possible values:
"left"
(default) - Displays the Toolbox on the left side of the design surface."right"
- Displays the Toolbox on the right side of the design surface."sidebar"
- Displays the Toolbox as an overlay on top of the Property Grid. Use thesidebarLocation
property to specify the Property Grid position.
- Type:
- toolboxLocationType readonly
- Implemented in:
- SurveyCreatorModel
Cancels the last change if possible.
- Type:
- () => void
- Implemented in:
- SurveyCreatorModel
- See also:
- redo * , onBeforeUndo
Unregisters value change event handlers for the specified properties.
- Type:
- (propertyNames: any, key?: string) => void
- Parameters:
-
propertyNames, type: any ,
An array of one or multiple property names.
key, type: string ,(Optional) A key of the registration that you want to cancel.
- Implemented in:
- Base
- See also:
- registerPropertyChangedHandlers
Uploads files to a server.
- Type:
- (files: {}, question: Question, callback: (status: string, data: any) => any, context?: { element: Base; item?: any; elementType?: string; propertyName?: string; }) => void
- Parameters:
-
callback, type: (status: string, data: any) => any ,
A callback function that indicates the upload status—"success" or "error"—as the first argument. If a file is uploaded successfully, the second argument contains the file's URL.
context, type: { element: Base; item?: any; elementType?: string; propertyName?: string; }
- Implemented in:
- SurveyCreatorModel
- See also:
- onUploadFile
Validates the property values of the focused element.
- Type:
- () => boolean
- Return Value:
-
true
if all property values of the focused element are valid or if no element is focused,false
otherwise.
- Implemented in:
- SurveyCreatorModel
- See also:
- onSelectedElementChanging * , onSelectedElementChanged
Copyright © 2024 Devsoft Baltic OÜ. All rights reserved.